In this blog, you’ll learn what pull requests are, how to effectively create them, how to give feedback on PRs, and how to respond to feedback.
What is a Pull Request (PR)?
A Pull Request is a request for changing code in a repository. Once you make the changes you need in your code, you submit a PR. Once submitted, interested parties perform a code review and provide you with any feedback or changes required.
Pull Requests are typically used by teams for shared collaboration and feature work or bug fixes. The idea is to make sure well written, high-quality code and bug-free code gets pushed to the repository.
How to Create a Pull Request
Breakdown Your Story/Feature
Before you start working on a story or feature, make a mental/written note on how you want to break it down into several smaller PRs. Smaller PRs don't just help your reviewer but also yourself as YOU can keep track of your progress and get frequent feedback. Another benefit is as the codebase is shared with other developers, reverting a change gets a lot easier. Therefore you want to try to keep the scope of your PR to a single issue while breaking it down.
For example, let’s say you’re implementing a to-do list and have the following story:
As a user, I can add and delete items to/from a list respectively
I would break it down by:
PR #1: Add a text box and an add button on the page.
PR #2: Clicking on add button adds the item to the list
PR #3: Clicking on delete button on the list item deletes that item from the list
You will get reviewer blessings 🙏 for doing so. Imagine if your co-worker blasted a HUGE PR, you wouldn’t want to review, would you? 🙈
Add Comments on Your PR
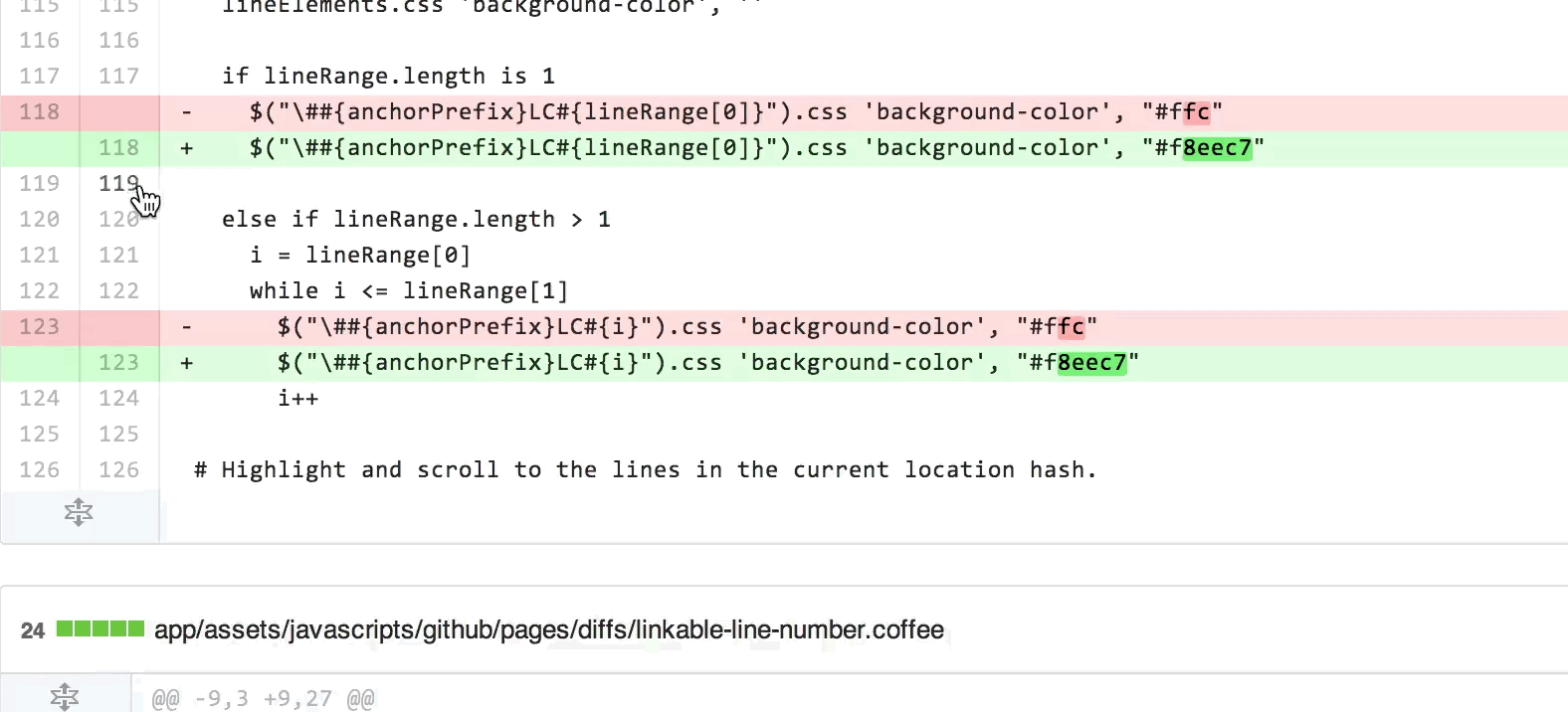
Wherever you have done something different, usually, it’s a good idea to 🔗 add a comment on the line number explaining why you did what you did. If you are not entirely sure of your code, you should tag “@” at those who can provide the most context on that code to get some feedback. For example,
You used a new function from the library Lodash called isNan which might not be used in the codebase a lot; you might want to add a comment there saying, "This function basically checks if the value is Nan and returns a boolean."
This helps your reviewer out and saves time, especially if they don't already know what the function does.
Add Screenshots/Gifs
Screenshots and gifs are proof that the code you submitted works! This increases the likelihood of acceptance and also gives your reviewer a visual of what you have implemented. A screenshot definitely works however when your code is doing more than what a screenshot can capture, create a gif. By using a gif, you can click around and show everything that your PR covers. My favourite one is: Licecap 🔗
Tests are Documentation of Your Code
Whenever you want to explain your code to other developers along with the intention of your changes. Don’t litter the code with code comments and ask yourself these questions to determine if it’s too difficult to understand:
- Can I split this code into smaller functions?
- Can I rename this function to something more obvious? Ask your team for function name suggestions if you like.
- How can I simplify my code further so it isn't as complex?
Irrespective of what you pick, you should write a test. 🔥
While your tests are there to test your logic, they're also documentation of your code. Try to add a test explaining that part of your code.
How to Review Pull Requests
Be Empathetic
As a reviewer, it’s essential to provide feedback politely. Remember that you are reviewing your teammates’ code who you like to talk to and go for team outings, lunches etc. **Feelings can get hurt easily!**💥 So, be empathetic during your reviews.
Make Yourself More Familiar with the Code
Always get in the habit of teaching, it doesn’t matter if you consider yourself a junior or a senior developer. Instead of telling them what the problem is, ask them questions, make them think and use a friendly tone. Here are a few examples:
- Do you think maybe we could assign this to a variable and re-use it on line 9?
- Could we possibly use this helpful utility that already does that for us which our dear teammate Sarah built?
- Can we move this code in its own function so we can write more tests 😃?
- What do you think about trying this option 🤔?
- I’m not sure if I understand the whole picture, could you explain what this function is doing? This might make them think the function name could be renamed. After they explain what it does, then follow it up with a suggestion such as:
“I get it now, sorry for being slow! I’m not sure but do you think we could rename this function to option1, option2 or something along those lines, what are your thoughts”?
Always ask them their thoughts, remember you are a reviewer, and they wrote the code so it's possible they have more context than you do.
Just imagine if your co-worker said something along the lines of, “Don’t do this, just rename the function”. That phrasing is much harsher and less friendly than the example above. It sounds like you're giving them a command rather than taking into account their input.
Always Provide Suggestions for Code Improvements
Rather than just telling them, “this code could be improved”. Provide them with specific suggestions/examples on how it could be improved further, such as:
This code works perfectly but, after I started reading, I thought of another idea that I wanted to run it by you. I’m not sure, but what about:
sample code #1,
sample code #2
What are your thoughts?
Use Emoji’s 😎
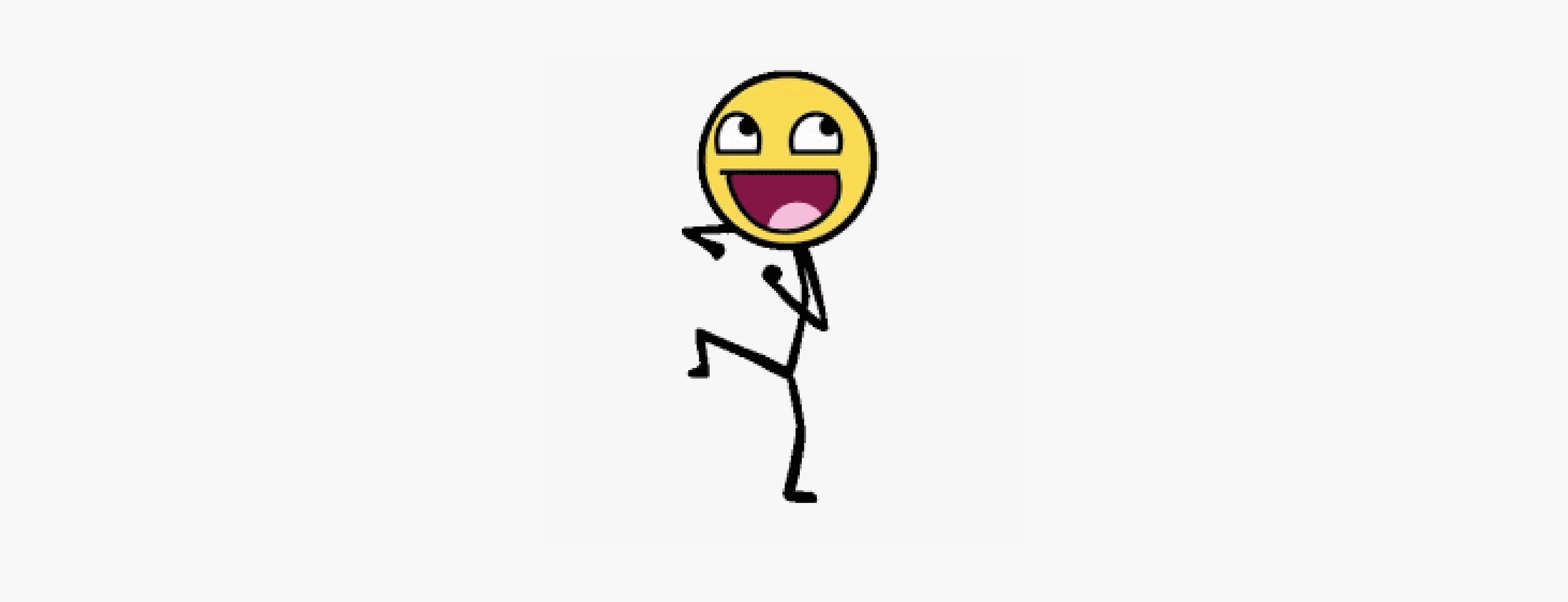
Online criticism is misinterpreted easily. That's why emojis are one of the best tools for improving your online communication. Using them automatically adds a friendly tone to your messages. You can use them when you start feeling that the conversation is getting too serious. But if that’s not your style then don’t force it, keep it real.
Take it Offline
If you find yourself going back and forth in a conversation with your teammate, it's getting too long or leading nowhere - take it offline. There is no point spending time typing when you can quickly hop on a call 🤙or walk 🚶 to their desk.
When you meet face to face, you automatically develop more empathy and understand their point of view even further and alternatively, they understand yours. When you come to a conclusion, post a comment on the PR summarizing your discussion so other readers following along are aware of it and your future self will thank you for it.
Nitpick
While reviewing code, you may find something that is a nitpick i.e. it may not necessarily block the approval of the PR but is something to consider. Nitpicks are not important but technically correct. They can be related to grammar corrections, unintentional new lines, aesthetics, minor code refactor and more.
For example,
- nit: extra white space
- nit: can we assign this value to a constant for better readability purposes?
How to Respond to Feedback
The rules that apply while submitting a PR also apply while responding as well.
When Suggestions are given
Always remember that you are writing code and collaborating. If a suggestion is given, always treat it as a possibility for improving your code. Make sure to followup if you don’t understand the suggestions given, ask for clarification. Here's an example,
“Can you please provide an example? or Can you please clarify further? I don’t fully understand what you mean.”
If you think the feedback is valid, implement it! However big or small the change is, if you think the feedback is valid, always reply and let the reviewer know that you took their feedback. For example,
"That's an excellent point, change coming right away!"
"Good point, thanks for catching that!"
If You Disagree 👈
Disagreements are bound to happen as different developers have different ways of writing code. Just remember to try to voice your opinion in a friendly manner. If you agree with them partially, then let them know but ensure you provide your reasoning for disagreeing. For example,
That's a great point. I agree with your first suggestion, I'll do that right away. Totally missed that one. Although for the 2nd one, I did consider that option and decided to not go that route as for the following reasons, we don't want to display that. // or **whatever your reasoning is, be honest. **👈
Make Your Commit Message Obvious
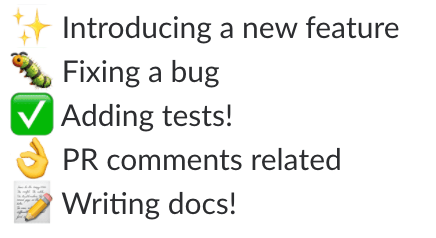
Now that you've spent time on your feedback, write a good commit message that lets the reviewer know you considered their feedback but don’t make the message too long. A very handy tool is 🔗 Gitmoji. 😍 They provide an easy way of identifying the purpose or intention of a commit just by looking at the emojis used.
Take it a Step Further
You've made changes in your code based on the feedback you received. Now if your code handles an additional scenario you weren't aware of, may have missed, there's your chance to “Write a test!”
Once your code is merged in, a defect gets filled. Once you fix that defect, add a test to make sure that the defect is actually fixed and there’s proof 🕵️. Now, it's documented!! 😃 You get brownie🍫 points for taking this extra step and will also earn the trust of your team.
I hope you enjoyed reading this article and learned something. If you did, please share your personal experience on pull requests in the comments. If you have any questions, please feel free to reach out on my Twitter.