
Image loading is an important job of many Native applications for iOS and Android. We want to load images as quickly as possible, and to load them only once.
Image
When writing apps with React Native you have access to the built-in Image component.
Image usage:
<Image source={{ uri: 'https://facebook.github.io/react/img/logo_og.png' }} />
React Native's Image component handles image caching like browsers for the most part. If the image is returned with a Cache-Control header than that will determine the caching behaviour. e.g. If the server returns an image with Cache-Control: max-age=365000000 (one year) than React Native shouldn't try to re-download the image for a year (although the cache does have a finite size). Even so, many people have noticed:
- Flickering.
- Cache misses.
- Low performance loading from cache.
- Low performance in general.
This has lead people to look for other solutions for image loading in React Native.
Existing Modules
There are some existing npm modules that attempt to solve the problems with Image. These modules implement caching logic in JS and store the images on the file system.
A problem I had with these modules is that they don't support adding headers to image requests, or stripping authorization tokens from query parameters. The result of this is that loading:
<Image source={{ uri: 'https://facebook.github.io/react/img/logo_og.png?token=tokenA' }} />
Then later loading:
<Image source={{ uri: 'https://facebook.github.io/react/img/logo_og.png?token=tokenB' }} />
Will result in a cache miss.
Aside from that, implementing the caching logic in JS is not optimal for performance. These solutions are also less well tested than existing Native image loading solutions.
FastImage
To solve these issues, I developed a new image component for React Native. FastImage is a performant replacement for React Native's Image component that more aggressively caches images.
FastImage (React Native) is a wrapper around SDWebImage (iOS) and Glide (Android). These modules have been around for years and are both widely used in production apps. They are performant and well tested.
With FastImage you can:
- Aggressively cache images.
- Add authorization headers.
- Prioritize images.
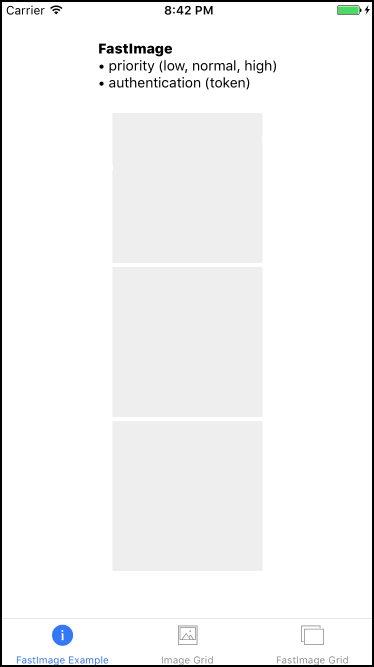
FastImage usage:
<FastImage
source={{
uri: 'https://facebook.github.io/react/img/logo_og.png',
headers:{ Authorization: 'someToken' },
priority: FastImage.PriorityNormal
}}
/>
Grab FastImage off npm and let me know how it works for you, or leave a comment if you have any thoughts on alternative caching strategies.
Learn More
If you're looking for more in-depth tutorials and learning opportunities, check out our various JavaScript training options. Better yet, contact us to inquire about how you can ramp up your knowledge of the fundamentals with custom course material designed and delivered to address your immediate needs.