Server Side Rendering (SSR)
Server Side Rendering (SSR) improvements in Angular is a common priority request and Angular 17 aims to bring SSR in line with other reputable frameworks. Introduced in version 16, hydration is production ready and doesn’t need to include setup. There is ESM support for server builds and speed improvements for server bundlers. The application builder now includes esbuild and Vite along with dependency injection debugging. Webpack builder is still supported but the Angular community is being nudged toward using the other two. Resumability also sees some improvements with this version. View the documentation on SSR in Angular which goes into greater detail on how hydration works.
View Transitions API
Angular 17 has opt-in-based support for the View Transitions API. It provides a mechanism for easily creating animated transitions between different DOM states while also updating DOM contents.
As a user navigates through the application, View Transitions API allows navigation bars, images, and other parts of the UI to animate smoothly between their visual states in completely separate HTML documents, helping users understand how they relate across the application.
You can read more about the View Transitions API here.
Declarative Control Flow Template Syntax
A new type of template syntax for structural directives is coming out. In the past, a developer conditionally rendering an element in html could use the *ngIf syntax, along with elseIf and else. One nuisance with this is you have to define the template for the other conditions elsewhere and reference them in the condition. Based on a preference poll by the Angular team, the @ symbol makes up the new syntax for conditional rendering. Now elements can be scoped inside of the condition they will appear in.
Unlike the *ng syntax, you don’t have to import anything to use the control flow syntax; it is now baked into Angular and pipes still function with the control flow syntax. Additionally, because it operates at a lower level, this results in performance improvements, mostly for loop logic, and it can identify when you swap two items in a list as part of the loop logic. These advantages result in an approximately 30% faster computation speed.
For the time being, this does not replace the old *ng syntax, you can use both.
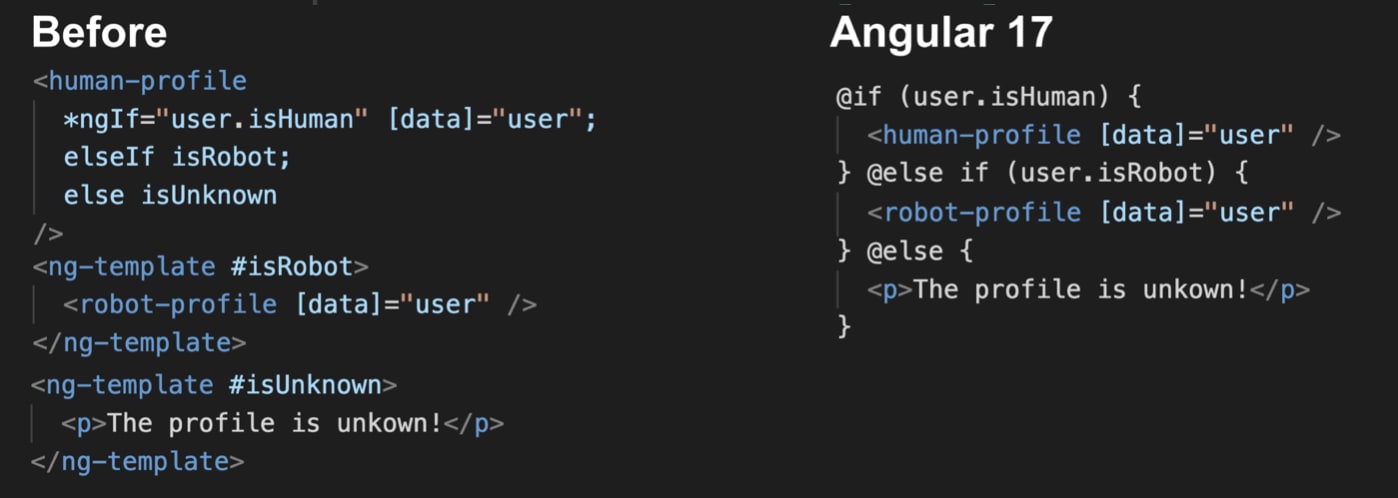
Furthermore, the trackBy function is now mandatory in the @for loop since developers often forgot to include in *ngFor.
Deferrable Views
Angular 17 introduces deferrable views that load and render template elements only when needed, enhancing application performance by reducing the simultaneous rendering of views. To maximize efficiency, focus on rendering critical page elements, such as videos before comments. Angular already supports lazy loading via lazy routes, but it can be complex.
The defer template syntax is in developer preview. But how does it know when to defer content? When the defer block is used on a template, the angular compiler collects all the dependencies within the block and afterwards, the compiler produces a set of dynamic imports and at runtime those dynamic imports are invoked when the trigger condition is satisfied.
These features are available in both Angular CLI and server-side rendering (SSR). The new primitive, {#defer}, handles lazy loading. Views are always rendered with their dependencies. Swap triggers include when for imperative conditions and on for declarative event conditions. Angular 17 also enables prefetching of anticipated resources for better performance.
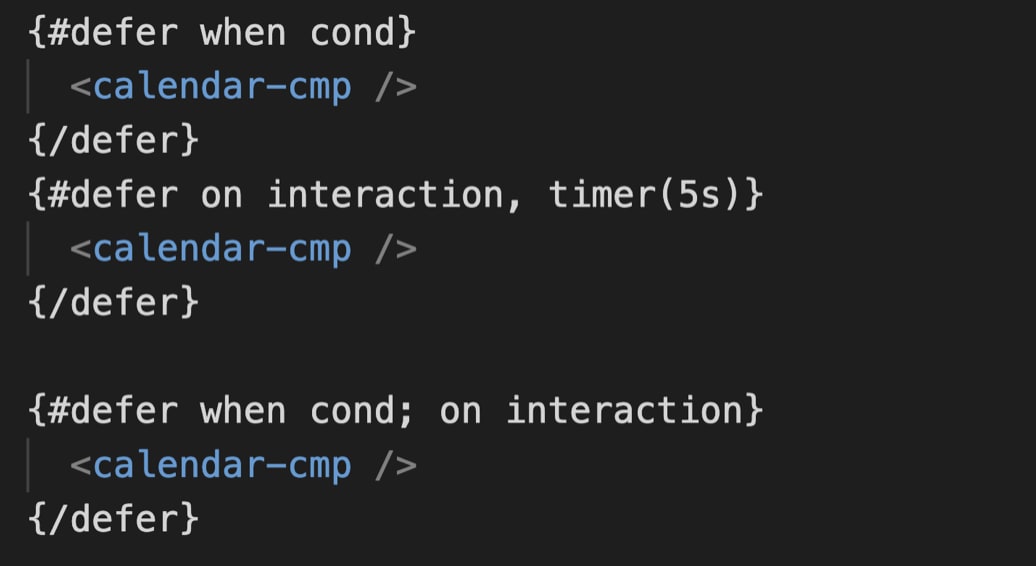
Signals
Signals, introduced in Angular 16 and becoming stable in 17, transforms the way you manage state and handle change detection. It offers a granular approach to state tracking, optimizing rendering updates across your application.
When using signals inside on push components, only components that have changed will be marked for check and then change detected.
These signals act as wrappers around data, making them highly versatile. Signals are characterized by two key components: a getter function for accessing the current value and a setter function for making modifications.
Angular Signals also introduces three primary reactive primitives:
- Signal: These are mutable and allow you to set, get, update, or reset them. Signals are accessed using getter functions, offering a straightforward way to manage component state.
- Computed: Computed primitives are read-only and derive their values from signals. They automatically run a function to update their value when the signals they depend on change, simplifying complex calculations.
- Effect: Effects are functions triggered by changes in specific signals, enabling you to manage side effects based on data updates. Angular tracks these dependencies, ensuring the right effects are executed when related signals change.
One significant advantage of Angular Signals is the enhancement of change detection. Traditionally, Angular developers avoid calling functions in templates because they rerun with every change detection cycle. Angular Signals addresses this issue by re-evaluating expressions only when a signal dependency changes. This optimization enhances performance and development flexibility.
Angular Signals can also replace the existing Observable design pattern, offering a more efficient approach to state management.
Notably, Angular Signals eliminates the need for Zone.js in change detection.
Additionally, there are application-level lifecycle hooks such as afterNextRender, afterRender, and afterRenderEffect. These hooks provide greater control over the rendering process. Aside from ngOnInit and ngOnDestroy, the other classic lifecycle hooks cannot be used with signals.
Signals, combined with other features of Angular 17, revolutionizes state management and change detection. It leverages reactive primitives, optimizes change detection, and provides advanced lifecycle hooks, making Angular development more efficient and flexible. View the angular documentation here.
CSS Removed Automatically
When components are destroyed, CSS relevant to that component will be removed from the DOM automatically along with it. Whereas previously, such CSS styling might remain in the DOM. When this feature is released, we can get a better look at how this is integrated and what the difference in performance will be.
ProvideRouter and RouterModule
With Angular 17, you can now use ProvideRouter and RouterModule to set up routes for testing. The following properties have also been moved to ProvideRouter and RouterModule.forRoot:
- paramsInheritanceStrategy
- urlUpdateStrategy
- canceledNavigationResolution
- malformedUriErrorHandler (moved to UrlSerializer.parse)
- titleStrategy
- urlHandlingStrategy
Upgrade to Node.js 18.13.0 Required
Angular 17 requires Node.js version 18.13.0 to operate.
Support for TypeScript(TS) 5.2
Angular 16 only supports up to TS version to 5.1, but this has been updated to allow for version 5.2 of TS and no lower than TS 4.9.3. New TS features include:
Faster Recursive Type Checking
Improvements on efficiency result in up to 40% faster checking for Recursive Types, such as the one below:
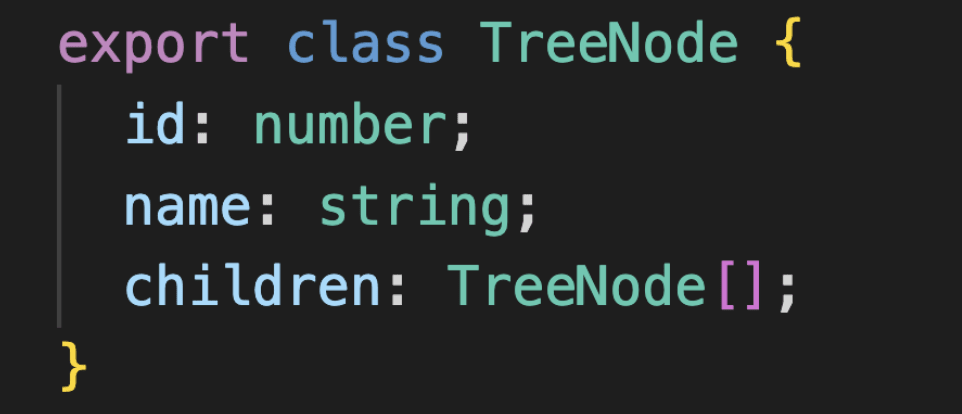
Improved Memory Leak Handling
TS 5.2 adds support for a new keyword ‘using’, a new EcmaScript addition. The ‘using’ keyword indicates resources should be closed or cleared when exiting scope. The images below illustrate the difference in how you would handle closing resources previously vs after version 5.2 with the ‘using’ keyword:
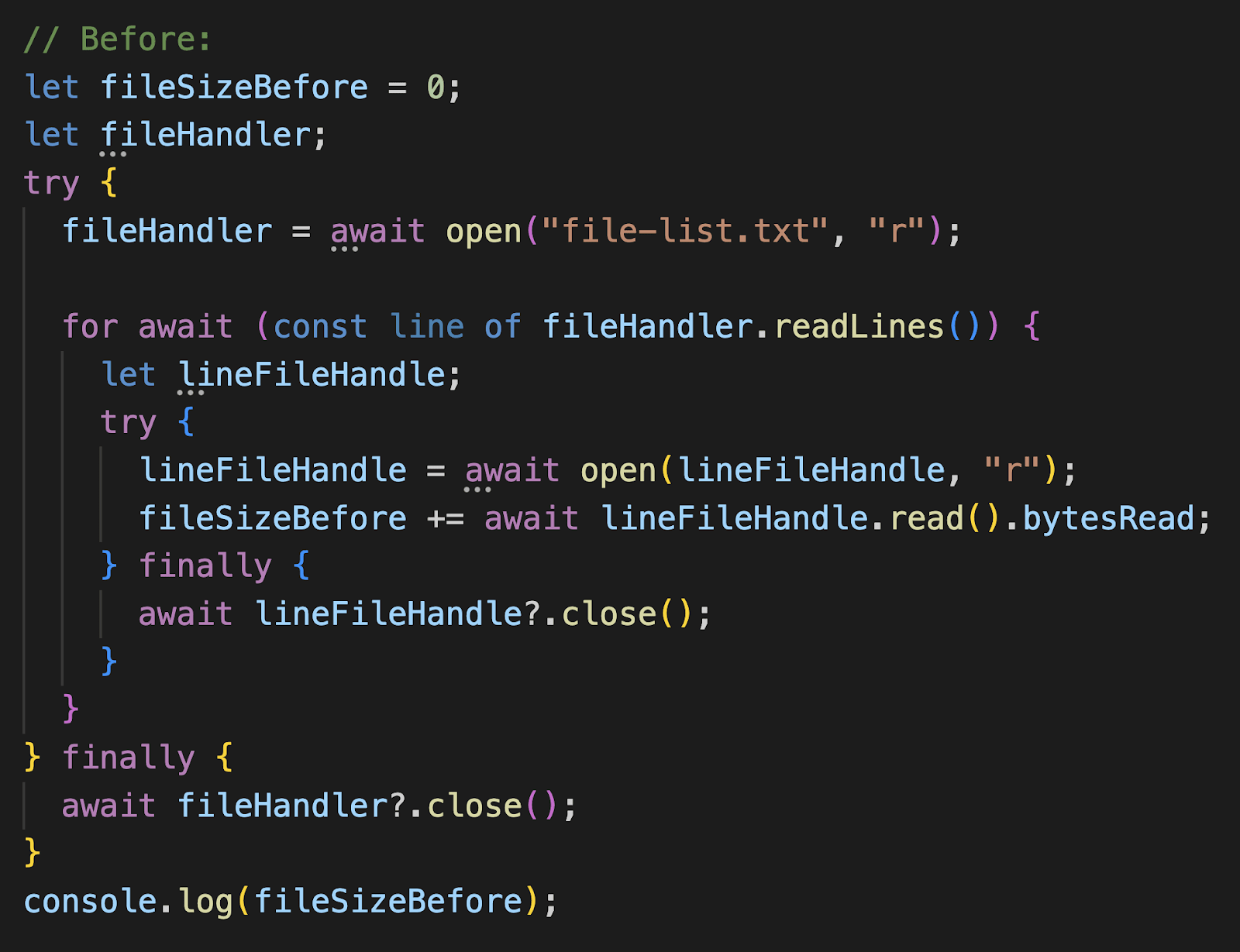
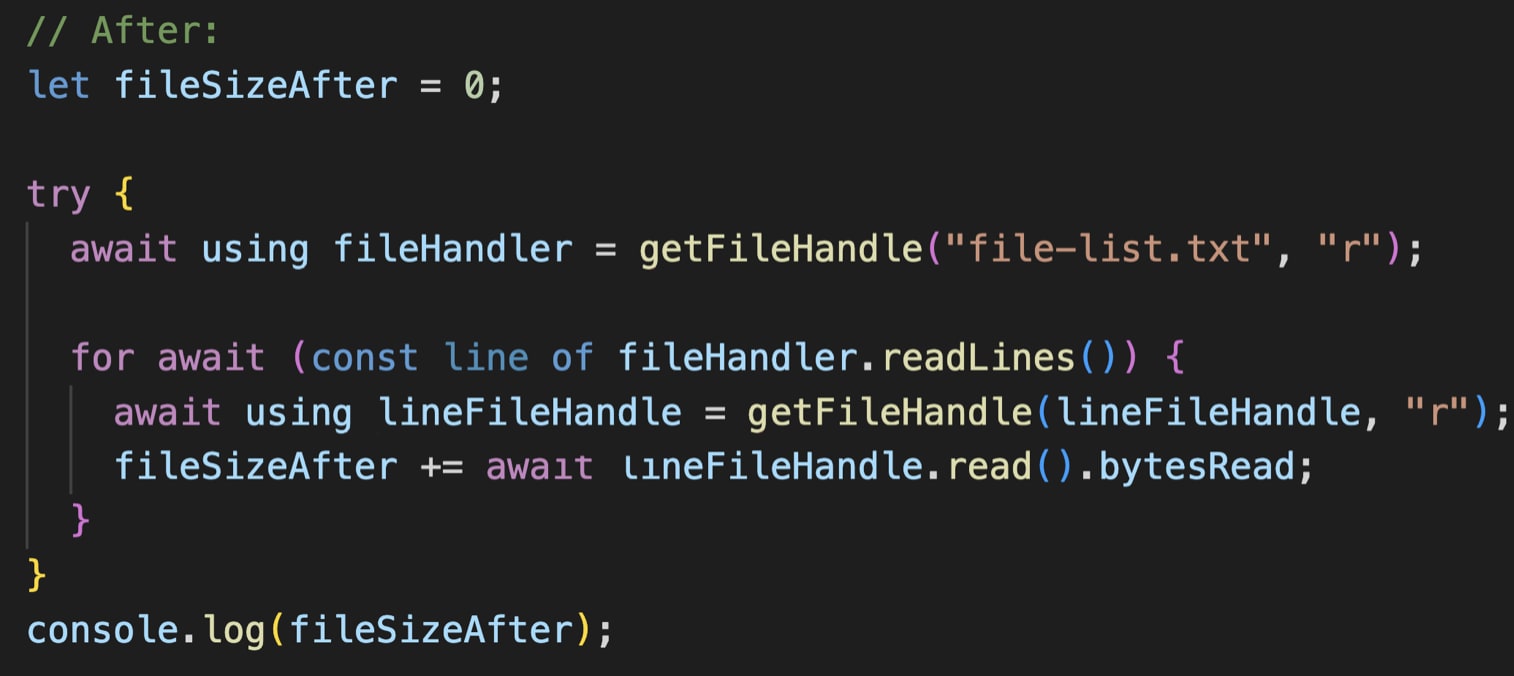
See more about explicit resource management for EcmaScript here.
Decorator Programming with Metadata now Supported
Decorators come with a Context object, but now support a metadata property within it, allowing serialization of the decorators.
Unions of Arrays
Example:
string [] | number [];
Declaring a variable or property as an array union can cause many breaking errors and headaches when using TS. Trying array functions involving such a union array, TS declares it doesn’t know if the desired array function exists on the array, since the resulting value could be one or the other. In 5.2, when such union arrays are attempted, TS will transform them behind the scenes.
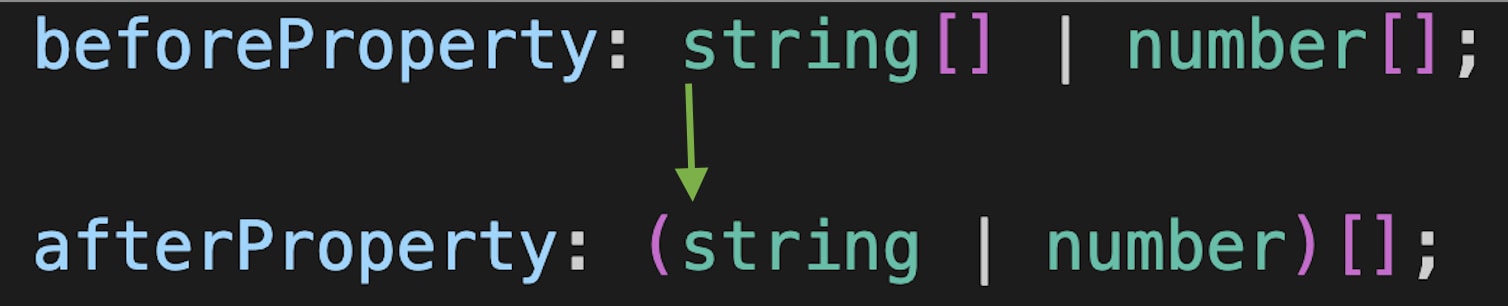
In this TS playground using v5.1.6, a union array produces an error on the filter method because TS doesn’t know which data type to use, whereas in this TS playground using v5.2.2, the same code works without complaints.
Copying Array Functions
A common objective is to copy an array and perform modifications on that copy.
However, simply writing the line below would modify the original array:
arr2 = arr1.sort((a, b) => a - b )
To help alleviate this issue, TS 5.2 supports a set of copying array functions, so you can safely make a new copy of an array, including a modification, without worrying about affecting the original.
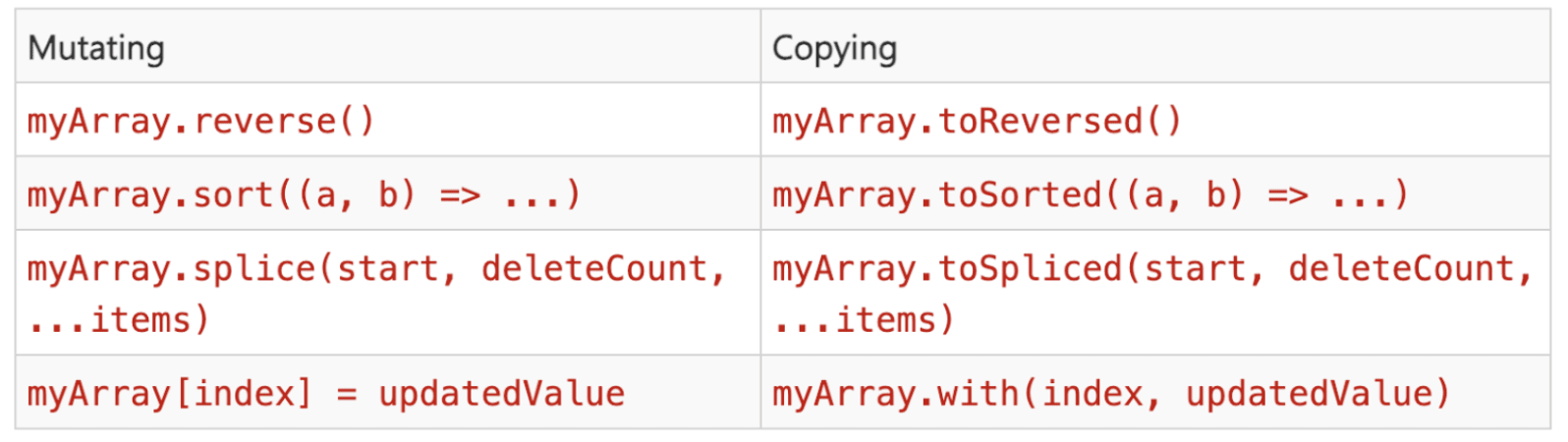
Example:
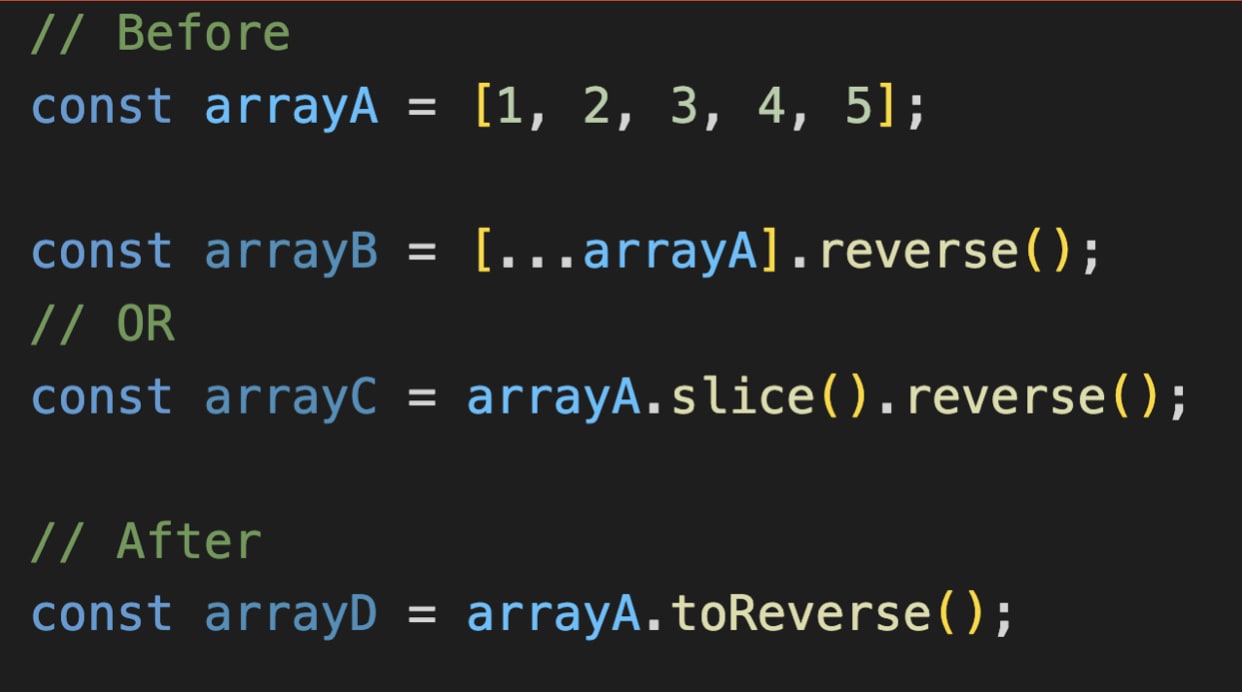
Comma Completion
Forgotten commas can now be automatically inserted.
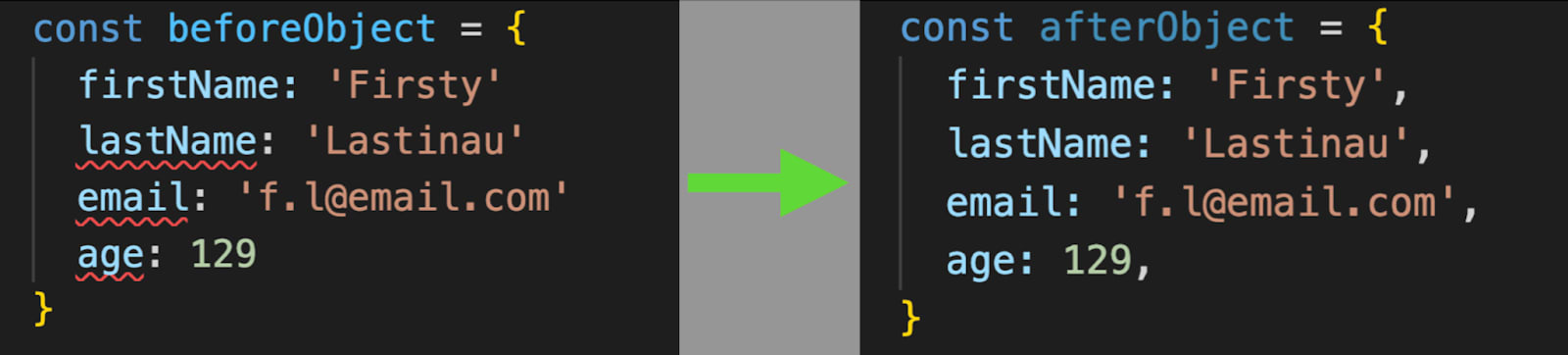
Conclusion
Angular 17 introduces a host of exciting features and improvements, from server-side rendering enhancements to the innovative View Transitions API, and a more efficient declarative control template syntax. Deferrable views offer performance benefits, while the Signals system transforms state management and change detection, eliminating the need for Zone.js and providing advanced lifecycle hooks. Additionally, the support for TypeScript 5.2 brings numerous enhancements, making Angular 17 a promising and powerful update for developers. These enhancements are set to significantly enhance the Angular development experience and provide more efficient, flexible, and performant solutions.
Special Angular Event
On November 6th, the Angular team gave a presentation on the upcoming features for version 17, most of which is already covered above. The areas they expand on and additional points made during the video event are detailed below.
New home for Angular development
Angular.dev is the new home for angular documentation and learning. To focus on developer experience, a new structure for documentation tutorials to help beginners learn Angular are included along with an essentials guide and an Angular playground where developers can test code in different versions. This new documentation setup is currently in beta. The classic documentation site is maintained for older versions but going forward, future documentation is being kept at Angular.dev. The new site will also support dark mode and the Angular team is embracing a new logo to fit with their philosophy of an Angular renaissance.
Other updates
- Standalone components are default now
- Can add standalone false flag when creating the project or component to make module based components
- Stylesheets property in components no longer necessarily an array
As developers using Angular become more familiar with the new features and more information rolls out, we're sure we’ll get a more complete view of all that Angular 17 has to offer.